20. Switch 语句
Switch
switch 语句和 if 语句非常相似。事实上,你可以编写一个程序,用 switch 语句完成和一系列 if-else 语句同样的事情。
那为什么还要用 switch 语句呢?因为 switch 语句执行速度一般更快,具体原因我们不在此详述。许多编程语言都有 switch 语句,包括 Java、Javascript、PHP、C++ 等等;Python 是个例外。
由于 Python 没有 switch 语句,我们将比较 C++ 中的一组 if-else 语句和 switch 语句。
点击图像放大。
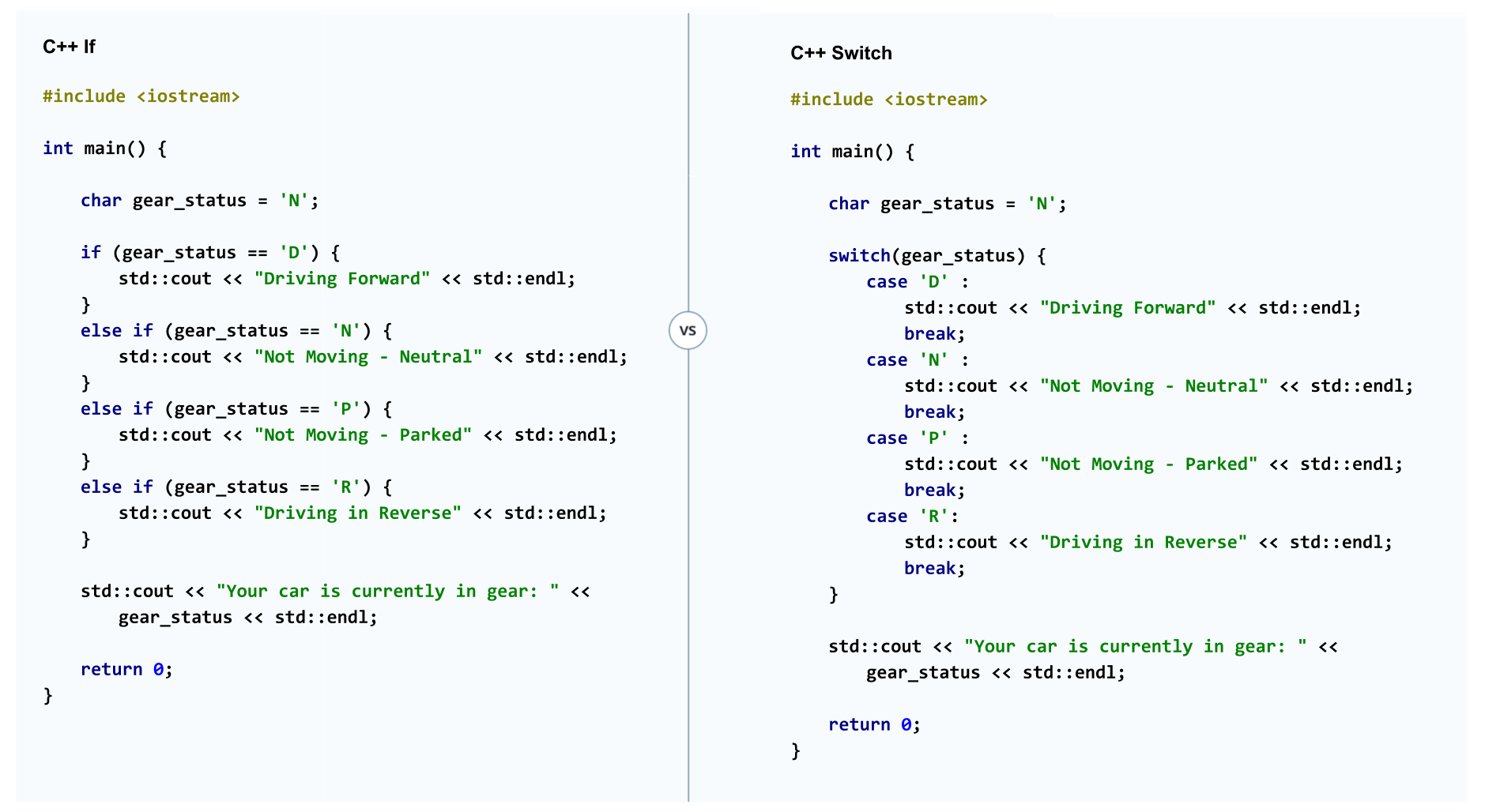
代码输出为:
Not Moving - Neutral
Your car is currently in gear:N
我们来分析一下 switch 语句中发生的事情:
```c++
char gear_status = 'N';
switch(gear_status) {
case 'D' :
std::cout << "Driving Forward" << std::endl;
break;
case 'N' :
std::cout << "Not Moving - Neutral" << std::endl;
break;
case 'P' :
std::cout << "Not Moving - Parked" << std::endl;
break;
case 'R':
std::cout << "Driving in Reverse" << std::endl;
break;
}
```
每次出现 case 时,代码都会检查 gear_status 变量是否与 case 相匹配。一旦 gear_status 找到匹配的 case, case 内的代码就会运行。
在 C++ 中,switch 语句功能是在匹配的情况下运行代码,然后运行下面所有 case。如果您希望代码在执行完匹配 case 后退出 switch,则需要对代码进行 分行 。
换句话说,如果代码编写时没有分行
# include <iostream>
int main() {
char gear_status = 'N';
switch(gear_status) {
case 'D' :
std::cout << "Driving Forward" << std::endl;
case 'N' :
std::cout << "Not Moving - Neutral" << std::endl;
case 'P' :
std::cout << "Not Moving - Parked" << std::endl;
case 'R':
std::cout << "Driving in Reverse" << std::endl;
}
std::cout << "Your car is currently in gear: " << gear_status << std::endl;
return 0;
}
代码还是跳过 'D' case, 但是,一旦代码与 “N” case 匹配,那么 “N”、“P” 和 “R” case 下的代码就会执行。
Switch 的限制
If-else 语句比 switch 语句更灵活。事实上,case 语句仅限于确定相等的整数。Switch 语句中的 case 也可以比较示例代码中的字符,因为 C++ 会将字符转换为整数。
switch 语句的一般形式如下所示:
int variable = integer;
switch(variable) {
case 1:
code statements;
break;
case 2 :
code statements;
break;
case 3:
code statements;
break;
case 4:
code statements;
break;
case etc ...
}
Switch 语句 - 游乐场
本游乐场部分,你可以练习编写 switch 语句。代码注释可以帮助你上手。您可以使用“测试运行”按钮运行你的代码,然后将你答案与 “solution.cpp” 进行比较。
Start Quiz:
//TODO Practice writing switch statements
// Don't forget an include statement if you want to use std::cout
int main() {
// TODO: write a program that outputs whether a vehicle is a motorcycle,
// 2-door coupe, 4-door car or a 5-door mini-van.
// You should create a variable that holds the number of doors in the vehicle
// A motorcycle would have doors = 0 for example.
// Then use a switch statement to output to the terminal the kind of vehicle
// you have
return 0;
}
//TODO Practice writing switch statements
// Don't forget an include statement if you want to use std::cout
#include <iostream>
int main() {
// TODO: write a program that outputs whether a vehicle is a motorcycle,
// 2-door coupe, 4-door car or a 5-door mini-van.
// You should create a variable that holds the number of doors in the vehicle
// A motorcycle would have doors = 0 for example.
// Then use a switch statement to output to the terminal the kind of vehicle
// you have
int doors = 5;
switch(doors) {
case 0:
std::cout << "Motorcycle" << std::endl;
break;
case 2:
std::cout << "Coupe" << std::endl;
break;
case 4:
std::cout << "Sedan" << std::endl;
break;
case 5:
std::cout << "Mini-van" <<std::endl;
break;
}
return 0;
}